Rich
Contents
Rich#
Rich is a library for rich - i.e. coloured, styled and formatted - printing to the console.
TLDR#
Just copy and paste this to the beginning of your code:
import rich
from rich import print # replace default print
from rich import print_json # pretty-print JSON
from rich import pretty
pretty.install() # add rich to the REPL
If you would like to record the terminal output, use the following:
from rich.console import Console
console = Console(record=True)
# console.log(...)
# console.print(...)
# console.print_json(...)
console.save_html('export.html')
Other Features#
Demo#
The following code
from rich.console import Console
console = Console(record=True)
# console message
console.log('This is a console.log() message\n')
# pretty printing
console.print("Let's define and print a dictionary 'd':")
d = {'a': 1, 'b': 2, 'c': list(range(3))}
console.print(f'd = {d}\n')
# JSON pretty printing
import json
console.print("And now let's convert it to JSON and pretty-print it")
console.print_json(json.dumps(d))
# export and save the console output as html
console.save_html('rich-demo.html')
will lead to output that looks like this:
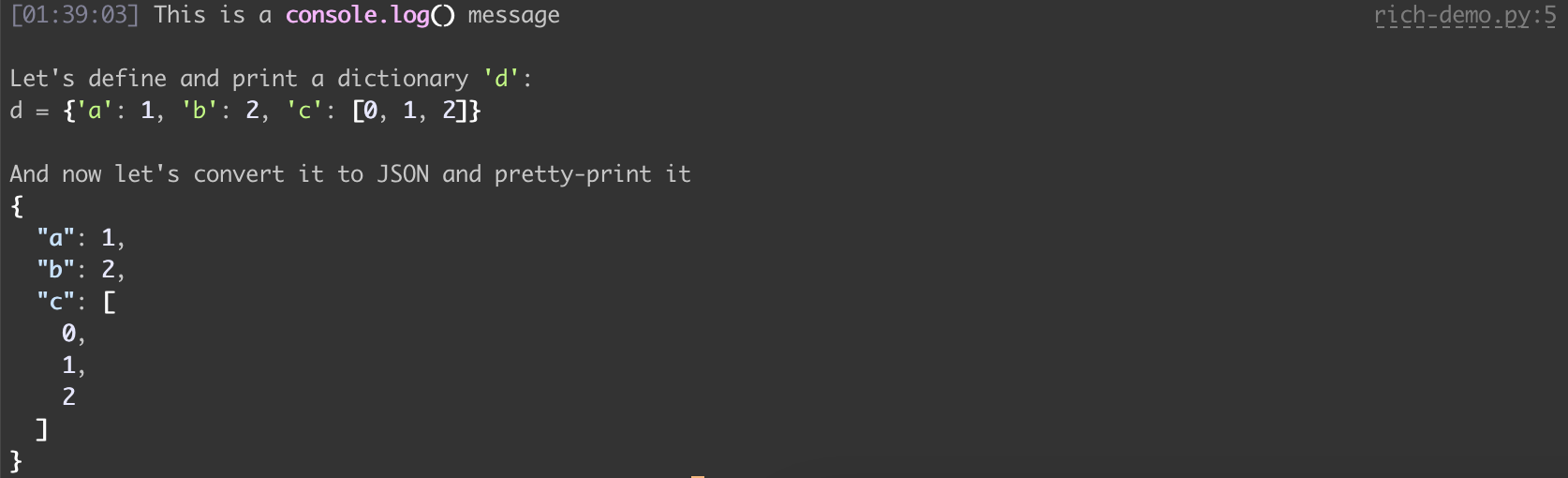
And it will also create a file rich-demo.html
that contains an export of the console as
HTML - which looks like this:
[01:39:03] This is a console.log() message rich-demo.py:5
Let's define and print a dictionary 'd':
d = {'a': 1, 'b': 2, 'c': [0, 1, 2]}
And now let's convert it to JSON and pretty-print it
{
"a": 1,
"b": 2,
"c": [
0,
1,
2
]
}